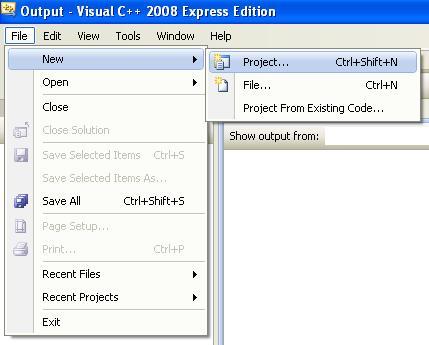
For an audio plugin or music application, creating a GUI is a challenging job for every programmer. Although there are lots of GUI Libraries / Frameworks available for developers to make the task easy.
For windows based applications, Windows API is Microsoft’s core set of application programming interfaces (APIs) available in the Microsoft Windows operating systems.
It provides the functionality to create and manage screen windows and most basic controls, such as buttons and scrollbars, receives mouse and keyboard input, and other functions associated with the GUI part of Windows.
You can use C, C++ compilers in order to compile the win32 application. Here in the following example, shows a simple win32 application for music programming, where first of all you have to create a window and add two simple buttons play and stop for your music player.
#include windows.h
const char g_szClassName[] = "musicWindowClass";
HINSTANCE g_hInst;
enum {
IDCC_BTN_PLAY,
IDCC_BTN_STOP
};
//Step 4: The Window Procedure
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM
wParam, LPARAM lParam)
{
switch(msg)
{
case WM_CREATE:
//Create simple play button
CreateWindow( "button
"Ok", // button text
WS_CHILD | WS_VISIBLE | BS_PUSHBUTTON |WS_TABSTOP,
194, 104, 88, 38, // x/y/w/h
hwnd, // parent
(HMENU) IDCC_BTN_PLAY, // button ID
g_hInst, // application instance handle
NULL);
//Create simple stop button
CreateWindow( "button
"Ok", // button text
WS_CHILD | WS_VISIBLE | BS_PUSHBUTTON | WS_TABSTOP,
294, 104, 88, 38, // x/y/w/h
hwnd, // parent
(HMENU) IDCC_BTN_STOP, // button ID
g_hInst, // application instance handle
NULL);
break;
case WM_COMMAND:
case IDCC_BTN_PLAY:
//Write your Music Play code here
break;
case IDCC_BTN_STOP:
//Write your Music Stop code here
break;
case WM_CLOSE:
DestroyWindow(hwnd);
break;
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hwnd, msg, wParam, lParam);
}
return 0;
}
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,
LPSTR lpCmdLine, int nCmdShow)
{
WNDCLASSEX wc;
HWND hwnd;
MSG Msg;
//Step 1: Registering the Window Class
wc.cbSize = sizeof(WNDCLASSEX);
wc.style = 0;
wc.lpfnWndProc = WndProc;
wc.cbClsExtra = 0;
wc.cbWndExtra = 0;
wc.hInstance = hInstance;
wc.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wc.hCursor = LoadCursor(NULL, IDC_ARROW);
wc.hbrBackground = (HBRUSH)(COLOR_WINDOW+1);
wc.lpszMenuName = NULL;
wc.lpszClassName = g_szClassName;
wc.hIconSm = LoadIcon(NULL, IDI_APPLICATION);
g_hInst=hInstance;
if(!RegisterClassEx(&wc))
{
MessageBox(NULL, "Window Registration Failed!", "Error!",
MB_ICONEXCLAMATION | MB_OK);
return 0;
}
// Step 2: Creating the Window
hwnd = CreateWindowEx(
WS_EX_CLIENTEDGE,
g_szClassName,
"The title of my window",
WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, 340, 120,
NULL, NULL, hInstance, NULL);
if(hwnd == NULL)
{
MessageBox(NULL, "Window Creation Failed!", "Error!",
MB_ICONEXCLAMATION | MB_OK);
return 0;
}
ShowWindow(hwnd, nCmdShow);
UpdateWindow(hwnd);
// Step 3: The Message Loop
while(GetMessage(&Msg, NULL, 0, 0) > 0)
{
TranslateMessage(&Msg);
DispatchMessage(&Msg);
}
return Msg.wParam;
}